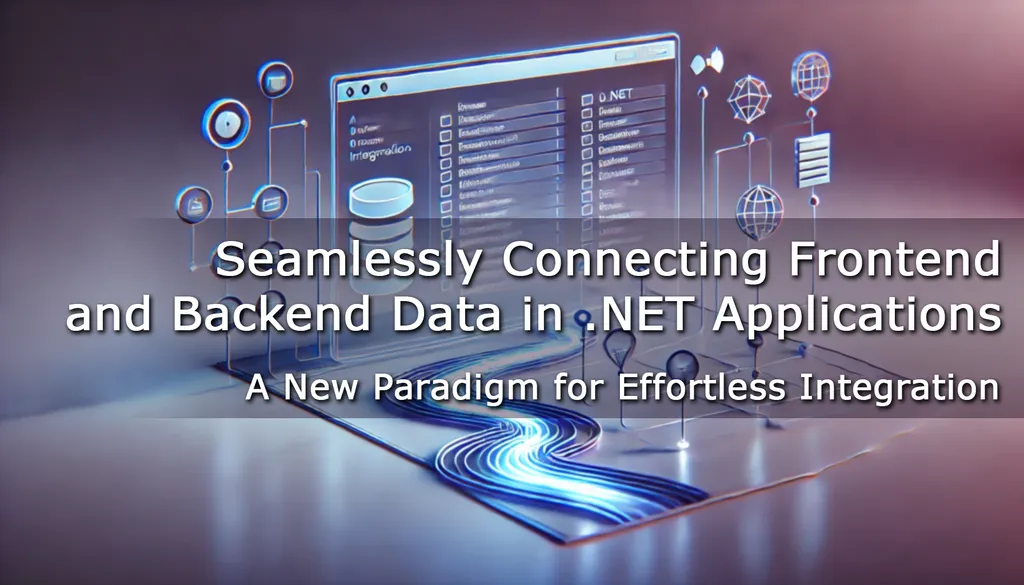
Seamlessly Connecting Frontend and Backend Data in .NET Applications: A New Paradigm for Effortless Integration
In today's fast‐paced software development world, engineers are often forced to write repetitive integration logic to connect user interfaces - especially data grids and tables - to their backend data sources. Every project, every new feature, and every iteration seems to reintroduce the same challenges: how do we reliably filter, sort, and display data without reinventing the wheel each time? The answer lies in automating these common tasks, thereby freeing developers to focus on business logic rather than boilerplate code.
The Problem: Repetitive Integration Logic
Consider a typical .NET application where you need to fetch, filter, and sort data from a SQL database using Entity Framework Core. In many projects, developers are repeatedly writing similar code snippets to achieve this functionality. For example:
// Example: Manually applying filters and sorting on a data source
public IQueryable<Person> GetFilteredAndSortedPeople(
ApplicationDbContext dbContext,
string name,
int? minAge,
string sortField
)
{
IQueryable<Person> query = dbContext.People.AsQueryable();
// Repetitive filtering logic:
if (!string.IsNullOrWhiteSpace(name))
{
query = query.Where(p => p.Name == name);
}
if (minAge.HasValue)
{
query = query.Where(p => p.Age >= minAge.Value);
}
// Repetitive sorting logic:
if (sortField == "Name")
{
query = query.OrderBy(p => p.Name);
}
else if (sortField == "Age")
{
query = query.OrderByDescending(p => p.Age);
}
return query;
}
Now, imagine this pattern repeated across multiple controllers, services, and even projects. Each time you need to tweak a filter or adjust a sort order, you are forced to manually update these code blocks - often duplicating logic and introducing room for inconsistencies or bugs.
Another example might involve converting query parameters from the UI into LINQ expressions:
// Example: Converting UI query parameters into LINQ filter expressions
public IQueryable<Product> FilterProducts(
IQueryable<Product> query,
Dictionary<string, string> filters
)
{
foreach (var filter in filters)
{
switch (filter.Key.ToLower())
{
case "category":
query = query.Where(p => p.Category == filter.Value);
break;
case "price":
if (decimal.TryParse(filter.Value, out var price))
{
query = query.Where(p => p.Price >= price);
}
break;
default:
break;
}
}
return query;
}
Problems with this approach:
- Wastes valuable time: Developers must repeatedly implement similar logic across different parts of an application.
- Increases error potential: Manual adjustments lead to inconsistencies and bugs.
- Reduces maintainability: Updates must be applied in multiple places, making maintenance a challenge.
Clearly, there is a need for a generic, reusable solution that abstracts away this repetitive integration logic.
Identifying the Requirements for a Generic Solution
To create a generic solution for handling data integration, certain core requirements must be met:
1. Serializability
For a solution to work seamlessly between the client and server, its filter and order definitions must be serializable. This allows easy transmission over HTTP without losing state.
2. Integration with IQueryable and EF Core
The solution must work directly with IQueryable
, ensuring that filtering and sorting logic is applied at the database level, improving performance.
3. Flexible Filter Definitions
A robust solution should support a variety of filtering operators such as:
- Equals (
=
) / Not Equals (!=
) - Greater Than (
>
) / Less Than (<
) - Contains (
=*
)
... and more.
4. Support for Overrides
Developers should be able to specify overrides for sorting and filtering logic to handle cases where default behavior isn't sufficient. For example, sorting should support custom overrides, like sorting Firstname
as [Firstname] [Lastname]
to ensure consistent ordering.
A Modern Approach to Automating Data Query Integration
An innovative solution was born: QueryLink. This library encapsulates all the above requirements into a single, easy-to-use package.
Key Features:
- Filter and Order Definitions: Define filters and sorting orders without writing complex LINQ expressions.
- Expression-based Overrides: Customize filtering or sorting logic using lambda expressions with type safety.
- Query String Conversion: Convert definitions to query strings for seamless transmission over
GET
parameters. - Direct IQueryable Integration: Ensures efficient query execution at the database level.
Getting Started: Hands-On Code Examples
Defining Filters and Sorting Orders
var definitions = new Definitions
{
Filters =
[
new("Name", FilterOperator.Eq, "John"),
new("Age", FilterOperator.Gt, 30)
],
Orders =
[
new("Name"),
new("Age", IsReversed: true)
]
};
Customizing with Overrides (Optional)
var overrides = new Overrides<Person>
{
Filter =
[
new(p => p.Name, p => p.FullName)
],
Order =
[
new(p => p.Name, p => p.FullName)
]
};
Applying Definitions to an IQueryable Source
IQueryable<Person> query = dbContext.People.AsQueryable();
query = query.Apply(definitions, overrides);
Embracing the New Way of Integration Logic
With QueryLink:
- Eliminate Repetitiveness: No more writing the same boilerplate code repeatedly.
- Improve Code Clarity: Declarative definitions make code easier to read and maintain.
- Enhance Consistency: Apply the same filtering and sorting logic across the application.
- Speed Up Development: Focus on business logic instead of integration logic.
Why QueryLink Is a Must-Have in Every .NET Project
QueryLink transforms UI-to-database integration by:
- Streamlining Development: Reducing the need for repetitive query logic.
- Ensuring Consistency: Standardizing filtering and sorting mechanisms.
- Enhancing Maintainability: Centralizing data integration logic.
Given these advantages, QueryLink is an essential library for every full-stack .NET application. By integrating this tool into your workflow, you empower your team to build more efficient and maintainable software.
Embrace the future of data integration with QueryLink.