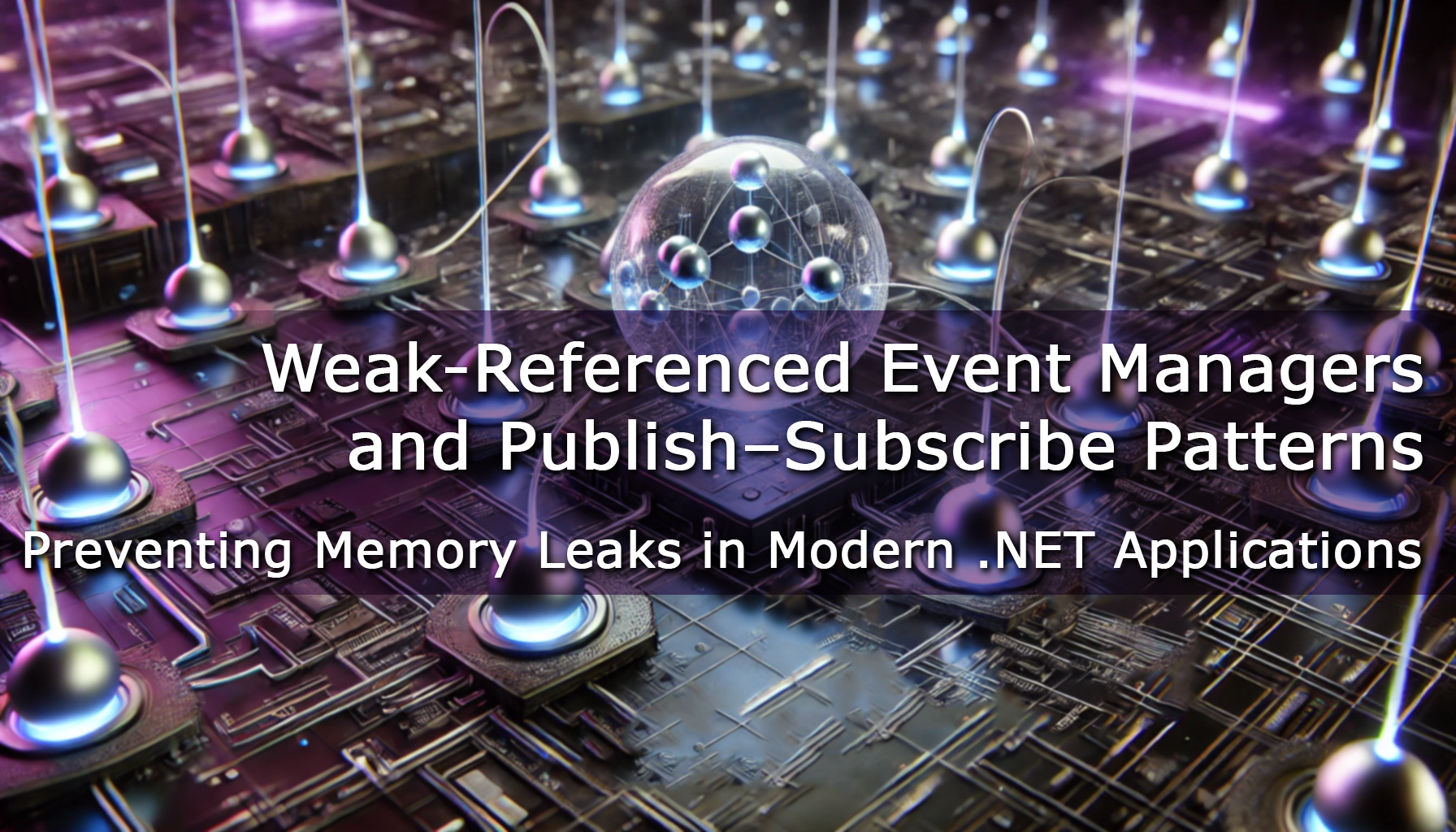
Harnessing Weak-Referenced Event Managers and Publish–Subscribe Patterns in .NET
In modern application development, event-driven programming is ubiquitous. Whether you're building rich desktop applications with WPF or web applications with Blazor, events provide a powerful way to decouple components. However, one common pitfall in event-based systems is memory leaks caused by lingering event subscriptions. The weak event pattern, along with publish–subscribe architectures, offers a robust solution by using weak references to hold subscribers. This ensures that objects can be garbage collected when no longer needed, even if they remain subscribed to events.
In this article, we will explore the theory behind weak-referenced event managers, demonstrate their practical implementation in .NET using C#, and illustrate how these concepts can be applied to real-world scenarios—including a look at the ByteAether.WeakEvent library.
Understanding the Weak Event Pattern
What Is a Weak Event?
In the typical event subscription model in .NET, the publisher holds a strong reference to each subscriber via its delegate invocation list. If a subscriber does not explicitly unsubscribe, it will remain in memory—even when it's no longer needed—causing memory leaks. The weak event pattern addresses this issue by holding references to subscribers weakly. In simple terms, a weak reference allows the garbage collector to reclaim the subscriber's memory if no other strong references exist.
According to Microsoft's documentation on WPF weak event patterns, the weak event pattern is particularly valuable in scenarios where the publisher's lifetime is longer than that of its subscribers. This pattern minimizes the risk of memory leaks by decoupling the event's lifecycle from its subscribers.
Key Benefits
- Memory Efficiency: By using weak references, subscribers do not prevent garbage collection, thereby reducing memory bloat.
- Decoupled Design: Publishers and subscribers can exist independently. This decoupling facilitates more maintainable code.
- Automatic Cleanup: There is less need for manual unsubscription, which reduces the risk of human error leading to memory leaks.
- Versatility: While particularly useful in UI frameworks (WPF, Blazor), the pattern is applicable in any event-driven architecture.
Deep Dive into .NET Garbage Collection and Weak References
The Role of the Garbage Collector
The .NET garbage collector (GC) is responsible for managing memory automatically. It frees objects that are no longer referenced by the application. However, in traditional event subscriptions, the publisher's reference to the subscriber via the event delegate prevents the GC from collecting the subscriber—even if the subscriber is no longer actively used.
How Weak References Work
A weak reference allows an object to be referenced without preventing its collection by the GC. Microsoft explains in its .NET garbage collection documentation on weak references that a weak reference object does not count as a "root" for garbage collection. This means that if the only references to an object are weak, the GC can reclaim that object's memory.
Example: Using WeakReference in C#
public class MySubscriber
{
public void HandleEvent(object sender, EventArgs e)
{
Console.WriteLine("Event handled by MySubscriber.");
}
}
public class Publisher
{
// List of weak references to event handlers
private List<WeakReference<EventHandler>> _handlers = new List<WeakReference<EventHandler>>();
public void Subscribe(EventHandler handler)
{
_handlers.Add(new WeakReference<EventHandler>(handler));
}
public void RaiseEvent()
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.TryGetTarget(out EventHandler handler))
{
handler.Invoke(this, EventArgs.Empty);
}
}
}
}
In this simple example, the Publisher
class holds a list of weak references to event handlers. When raising the event, it attempts to invoke only those handlers that are still alive. This strategy prevents dead objects from keeping themselves in memory alive indefinitely.
The Publish–Subscribe Pattern and Its Synergy with Weak References
The publish–subscribe (pub–sub) pattern is an asynchronous messaging paradigm where publishers emit events and subscribers listen for those events. This decoupling means that the publisher does not need to know the specifics of its subscribers. Traditionally, this pattern improves scalability and maintainability, particularly in distributed systems and event-driven architectures.
Combining Weak References with Pub–Sub
By integrating weak references into a publish–subscribe system, developers can manage event subscriptions more safely. The use of weak references ensures that even if a subscriber forgets to unsubscribe, the system remains robust against memory leaks. This is especially critical in long-running applications or dynamic environments like modern web frameworks.
Example: A Weak-Referenced Publish–Subscribe System in C#
Consider a more structured implementation where a mediator handles event subscriptions:
public interface IEventSubscriber<TEventArgs>
{
void OnEvent(object sender, TEventArgs e);
}
public class WeakEventManager<TEventArgs> where TEventArgs : EventArgs
{
private List<WeakReference<IEventSubscriber<TEventArgs>>> _subscribers
= new List<WeakReference<IEventSubscriber<TEventArgs>>>();
public void Subscribe(IEventSubscriber<TEventArgs> subscriber)
{
_subscribers.Add(new WeakReference<IEventSubscriber<TEventArgs>>(subscriber));
}
public void Unsubscribe(IEventSubscriber<TEventArgs> subscriber)
{
_subscribers.RemoveAll(wr =>
{
if (wr.TryGetTarget(out IEventSubscriber<TEventArgs> target))
{
return target.Equals(subscriber);
}
return true; // Clean up dead references
});
}
public void RaiseEvent(object sender, TEventArgs e)
{
_subscribers.RemoveAll(wr => !wr.TryGetTarget(out _)); // Cleanup
foreach (var weakSubscriber in _subscribers)
{
if (weakSubscriber.TryGetTarget(out IEventSubscriber<TEventArgs> subscriber))
{
subscriber.OnEvent(sender, e);
}
}
}
}
In this code, the WeakEventManager<TEventArgs>
class encapsulates the publish–subscribe pattern. It leverages weak references to avoid preventing the garbage collection of its subscribers. The cleanup logic ensures that any references to objects that have been collected are removed.
Real-World Applications: ByteAether.WeakEvent NuGet Library
The ByteAether.WeakEvent NuGet library provides a ready-to-use implementation of the generic weak event pattern using the publish–subscribe mechanism. It is built on the principles discussed above and is tailored for .NET developers seeking an out-of-the-box solution for managing event lifecycles.
According to its description:
The weak event pattern is a proven approach to managing event subscriptions in .NET, as detailed in Microsoft's documentation on WPF weak event patterns. This design avoids memory leaks by holding event subscribers with weak references, which means that even if an object remains subscribed to an event, it can still be garbage collected when no longer in use.
Leveraging the power of .NET's weak reference mechanism, as explained in the .NET garbage collection documentation, the WeakEvent library ensures that event subscribers do not prevent the garbage collector from reclaiming memory. This decoupling of publishers and subscribers provides a robust solution for managing event lifecycles without the need for manual unsubscription.
Benefits in Blazor and Other Modern Frameworks
When developing Blazor components, for example, unsubscribing from events during component disposal can be tricky. The ByteAether.WeakEvent library addresses this by ensuring that subscriptions are weak; once a component is no longer rendered, the weak reference does not prevent its memory from being reclaimed. This automatic cleanup is invaluable in preventing the accumulation of "dead" components that can degrade performance over time.
Example Usage in a Blazor Component
@code {
[Inject]
protected readonly Publisher _publisher { get; set; } = default!;
protected override void OnInitialized()
{
// Assume Publisher has a public property WeakEvent<MyEventData> OnPublish
_publisher.OnPublish.Subscribe(OnEvent);
}
public void OnEvent(MyEventData eventData)
{
// Handle the event (e.g., update UI state)
Console.WriteLine("Event received in Blazor component.");
}
public void Dispose()
{
// No need to manually unsubscribe, the weak reference handles cleanup.
}
}
In this Blazor example, the component subscribes to event in a Publisher
instance, that makes use of ByteAether.WeakEvent library. Even if the component is disposed, the weak reference does not hinder the garbage collection process, eliminating one of the common pitfalls in event-driven UI development.
Broader Implications and Cross-Platform Benefits
Although our examples and discussion have been centered on C# and .NET, the challenges of managing event lifecycles and preventing memory leaks are common across all programming environments. Languages and frameworks that use event-driven paradigms—such as JavaScript, Java, and even C++—face similar issues when subscribers outlive their useful life due to lingering references.
Applying Weak-Referenced Event Patterns in Other Environments
Many modern frameworks provide mechanisms similar to .NET's weak references. For instance:
- JavaScript: Libraries like RxJS help manage subscriptions in a reactive programming model. Although JavaScript does not have a built-in weak reference mechanism in all runtimes, the concept of unsubscribing (or using operators like
takeUntil
) plays a similar role in managing memory. - Java: The use of
WeakReference
in Java follows similar principles, allowing developers to build event systems where the publisher does not inadvertently extend the lifetime of the subscriber. - C++: With careful design, smart pointers and observer patterns can mimic weak event management to avoid memory leaks.
Thus, while the syntax and APIs differ, the fundamental benefit remains the same: decoupling the event subscription mechanism from the object lifecycle leads to more robust, maintainable, and memory-efficient applications.
Conclusion
The weak event pattern combined with the publish–subscribe model represents a powerful approach to managing events in software engineering. By utilizing weak references, developers can mitigate common pitfalls such as memory leaks and dangling subscriptions, ensuring that components are cleaned up promptly by the garbage collector. In the .NET world, where managed memory and automatic garbage collection are key features, these patterns are especially valuable.
Libraries like ByteAether.WeakEvent encapsulate these best practices, providing developers with a plug-and-play solution to implement weak event patterns easily. Whether you're developing desktop applications with WPF, building modern web applications with Blazor, or designing systems in other environments, understanding and leveraging weak-referenced event managers can significantly enhance application reliability and performance.
As the landscape of software development evolves—with more dynamic and component-based systems emerging—the need for robust event management becomes increasingly critical. Embracing patterns that promote decoupling and automatic resource management not only leads to cleaner code but also results in applications that are more scalable and resilient in the face of growing complexity.
By integrating these approaches into your development practices, you are better equipped to build systems that manage resources intelligently, ultimately leading to a smoother user experience and more maintainable codebases.