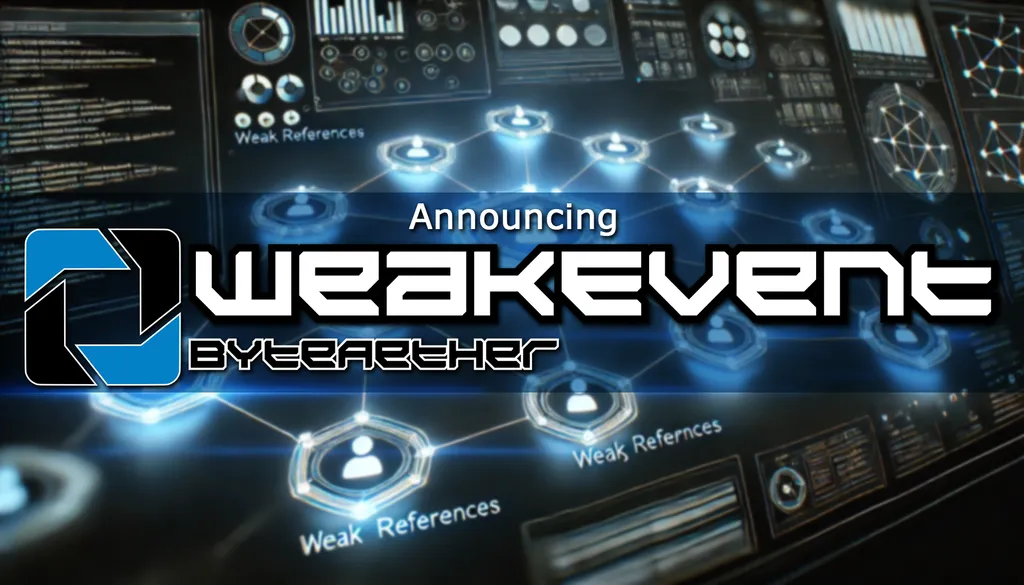
Announcing ByteAether.WeakEvent v1.0.0
We are thrilled to announce the release of ByteAether.WeakEvent v1.0.0, a NuGet library designed to revolutionize event management in .NET. This release marks the culmination of months of development, testing, and community feedback. With a focus on memory safety and ease-of-use, ByteAether.WeakEvent empowers software engineers and architects to build decoupled, maintainable, and high-performance applications without the pitfalls of memory leaks caused by traditional event subscriptions.
Tackling a Common Challenge in .NET
In modern .NET applications, event-driven programming is ubiquitous. Whether you are developing rich desktop experiences with WPF, dynamic web applications with Blazor, or any event-based system, managing event subscriptions efficiently is critical. A common challenge is that the standard event subscription model holds strong references to subscribers, often leading to unintended memory retention and, ultimately, memory leaks.
The weak event pattern offers a solution by holding references to event subscribers weakly. In this model, the garbage collector can reclaim subscriber memory even when they remain subscribed to an event, provided no other strong references exist. This release of ByteAether.WeakEvent brings this pattern to your fingertips with a clean API, automatic cleanup of defunct subscriptions, and a robust publish–subscribe mechanism tailored for .NET applications.
For more details on the concepts behind weak events and publish–subscribe patterns, refer to our in-depth article on the subject. You can also check out the GitHub repository for source code and additional documentation.
Understanding the Weak Event Pattern
What Is a Weak Event?
In conventional .NET event handling, the publisher holds a strong reference to each subscriber through its delegate list. If subscribers are not explicitly removed, these references persist, keeping objects in memory even after they are no longer needed. This is the primary cause of memory leaks in event-driven systems.
A weak event solves this by holding subscribers via weak references. A weak reference permits the garbage collector to reclaim an object's memory if no other strong references exist. This behavior is especially useful in scenarios where the publisher has a longer lifetime than its subscribers.
The Theory Behind Weak References
As explained in foundational materials on weak event management:
- Memory Efficiency: Weak references ensure that an object is not kept alive solely by its event subscription.
- Decoupled Design: Publishers and subscribers can evolve independently, fostering better modularity.
- Automatic Cleanup: There is no need to worry about manually unsubscribing every time a component is disposed. The weak reference mechanism handles cleanup, thereby reducing the risk of human error.
Consider this simplified code example that demonstrates the essence of weak references in event handling:
public class Publisher
{
// List of weak references to event handlers
private List<WeakReference<EventHandler>> _handlers = new();
public void Subscribe(EventHandler handler)
{
_handlers.Add(new WeakReference<EventHandler>(handler));
}
public void RaiseEvent()
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.TryGetTarget(out EventHandler handler))
{
handler.Invoke(this, EventArgs.Empty);
}
}
}
}
In this model, event subscribers are not held strongly, which allows the garbage collector to reclaim memory for any subscriber that is no longer in use.
Key Benefits of ByteAether.WeakEvent
Memory Efficiency and Automatic Cleanup
One of the primary advantages of using ByteAether.WeakEvent is its ability to manage memory efficiently. By storing subscribers as weak references, the library ensures that objects are not unnecessarily held in memory. This is particularly beneficial for applications with long lifetimes, where small memory leaks can accumulate over time and degrade performance.
Simplified Event Management
Traditionally, developers must be vigilant in manually unsubscribing events—an often error-prone process. ByteAether.WeakEvent automates the cleanup process by removing dead references whenever an event is raised. This simplifies event management, reduces boilerplate code, and minimizes the risk of memory leaks.
Versatility Across Application Types
The library is designed to be flexible:
- Events With or Without Data: Whether you need to send event data or simply signal that an event has occurred, ByteAether.WeakEvent provides a straightforward API for both scenarios.
- Support for Asynchronous Patterns: With native support for asynchronous publishing, the library is well-suited for modern application architectures that leverage async/await patterns.
- Ideal for UI Frameworks: In frameworks like Blazor, where component lifecycles can be complex, the library's weak reference management ensures that components are garbage collected promptly after disposal.
Exploring the API and Usage Examples
Simple Event Subscription
For events that do not require additional data, the API is as simple as subscribing a handler and publishing the event:
using ByteAether.WeakEvent;
// Create an instance of the weak event without event data
var myEvent = new WeakEvent();
// Create a subscriber and subscribe
var subscriber = () => Console.WriteLine("Event received!");
myEvent.Subscribe(subscriber);
// Raise the event
await myEvent.PublishAsync();
In this example, the handler is invoked if its target is still alive. Dead subscribers are automatically pruned from the subscription list when the event is raised.
Event Subscription with Data
When you need to transmit data along with the event, the WeakEvent<TEvent>
class comes into play:
using ByteAether.WeakEvent;
// Create an instance of the weak event with event data
var myEvent = new WeakEvent<MyEventData>();
// Create a subscriber and subscribe
var subscriber = (MyEventData data) => Console.WriteLine("Received: " + data.Message);
myEvent.Subscribe(subscriber);
// Raise the event with data
await myEvent.PublishAsync(new MyEventData("Hello, World!"));
// Define your event data
public record MyEventData(string Message);
This approach allows your events to be rich in context, with subscribers receiving any necessary data without compromising the integrity of the memory management system.
How ByteAether.WeakEvent Works Under the Hood
The Publish–Subscribe Paradigm
At its core, ByteAether.WeakEvent embraces the publish–subscribe model, a design pattern that decouples the event publisher from its subscribers. In this architecture:
- Publishers emit events without any knowledge of which components are listening.
- Subscribers listen for events and react accordingly, without the need for explicit deregistration.
This decoupling not only simplifies code but also enhances scalability and maintainability—attributes that are critical in both desktop and web applications.
Detailed Implementation Insights
The library leverages the built-in .NET WeakReference<T>
to hold event subscribers. When an event is published:
- It iterates through the list of weak references.
- Attempts to retrieve the target object for each subscriber.
- Invokes the event handler only if the target is still alive.
- Cleans up any references that have been collected by the garbage collector.
This strategy ensures that your application's memory footprint remains minimal, as there is no lingering of obsolete event handlers. Detailed examples and explanations can be found in our documentation and our in-depth article on the weak event pattern.
Real-World Applications and Scenarios
UI Development with Blazor and WPF
In UI frameworks such as Blazor and WPF, the lifecycle management of components is crucial. Consider a Blazor component that subscribes to events during its initialization:
@code {
[Inject]
protected readonly Publisher _publisher { get; set; } = default!;
protected override void OnInitialized()
{
_publisher.OnPublish.Subscribe(OnEvent);
}
public void OnEvent(MyEventData eventData)
{
Console.WriteLine("Event received in Blazor component.");
}
public void Dispose()
{
// No need to manually unsubscribe, the weak reference handles cleanup.
}
}
In this scenario, even if a component is disposed, its subscription does not prevent garbage collection. This is a critical advantage in long-running applications where manual unsubscription could be easily overlooked, leading to potential memory leaks.
Server-Side and Distributed Systems
For backend services and distributed systems, resource management is paramount. ByteAether.WeakEvent's ability to automatically manage event subscriptions means that your server-side applications can scale more reliably. When objects are no longer in use, they are automatically cleaned up, reducing the overhead associated with manual memory management and ensuring smoother performance.
Cross-Platform Benefits
While our focus is on .NET, the principles behind weak event management apply broadly across programming environments. Whether you are working with JavaScript's reactive libraries, Java's weak references, or even observer patterns in C++, the core concept remains the same: decoupling event publishers from subscribers results in more resilient and maintainable systems.
Getting Started with ByteAether.WeakEvent
Installation Made Easy
The ByteAether.WeakEvent library is available as a NuGet package. To install, simply run:
dotnet add package ByteAether.WeakEvent
For those who wish to experiment with the latest features, you can also specify a preview version using the --version
option.
Documentation and Community
For comprehensive documentation, API details, and additional usage examples, please visit the GitHub repository. We encourage contributions and feedback from the community. If you encounter any issues or have suggestions, feel free to open an issue or submit a pull request.
A New Era of Event Management in .NET
ByteAether.WeakEvent v1.0.0 sets a new standard for event handling in .NET. By embracing the weak event pattern, the library not only addresses common pitfalls like memory leaks but also simplifies your codebase, allowing you to focus on building great features. Whether you are developing desktop applications, dynamic web apps, or large-scale distributed systems, this library is designed to make your event-driven programming more efficient, robust, and maintainable.
We invite software engineers and architects to integrate ByteAether.WeakEvent into their projects and experience the benefits of a lean, memory-safe event management system. Explore the GitHub repository and get started today!
Happy coding!