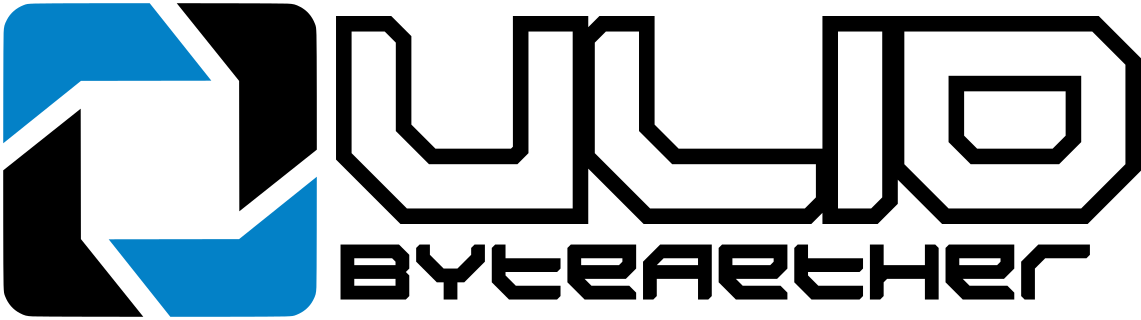
Announcing ByteAether.Ulid v1.0.0: A High-Performance .NET ULID Library for Modern Applications
Frustrated with GUIDs that aren’t sortable or human-readable? Meet ByteAether.Ulid - a ULID library designed to simplify your .NET development.
We’re excited to introduce ByteAether.Ulid v1.0.0, a high-performance .NET library for generating and working with ULIDs (Universally Unique Lexicographically Sortable Identifiers).
For developers managing distributed systems or working with time-sensitive data, ULIDs offer the perfect blend of uniqueness, readability, and sortability. ByteAether.Ulid takes it further by delivering exceptional performance, seamless integration with modern .NET projects, and comprehensive reliability.
Why ULIDs, and Why ByteAether.Ulid?
ULIDs provide unique identifiers that are:
- Globally Unique: No risk of collisions, even in distributed systems.
- Sortable: Lexicographically ordered, making them ideal for time-based sorting.
- Human-Readable: Unlike GUIDs, ULIDs use a base32 encoding that’s easy to interpret.
ByteAether.Ulid goes beyond standard implementations:
Feature | GUIDs | Integer IDs | ULIDs | ByteAether.Ulid Enhancements |
---|---|---|---|---|
Global Uniqueness | ✅ Yes | ❌ No | ✅ Yes | ✅ Yes |
Time-Based Sortability | ❌ No | ✅ Yes | ✅ Yes | ✅ Yes |
Human-Readable Format | ❌ No | ✅ Yes | ✅ Yes | ✅ Yes |
Overflow Handling | ❌ N/A | ❌ N/A | ❌ Sometimes | ✅ Graceful & Reliable |
ByteAether.Ulid fully adheres to the ULID specification while addressing common pitfalls, such as random-part overflow, ensuring a truly error-free experience.
Key Features of ByteAether.Ulid
1. Error-Free ULID Generation
Handles edge cases gracefully, like random-part overflow, by incrementing timestamps to maintain monotonicity without errors.
2. High-Performance Design
ByteAether.Ulid is optimized for speed and low memory usage, outperforming other libraries like NetUlid, NUlid, and Cysharp’s Ulid.
3. Seamless .NET Integration
- Supports .NET versions from .NET Standard 2.0 to .NET 9.0.
- Native support for ASP.NET Core route parameters.
- Fully compatible with
System.Text.Json
serialization and deserialization.
4. Versatile Conversion Methods
Effortlessly convert ULIDs to and from:
- Byte arrays
- GUIDs
- Strings
Getting Started
Installation
Getting started is as simple as installing the NuGet package:
dotnet add package ByteAether.Ulid
Run dotnet list package
to verify the installation. Visit the NuGet page for more details.
Usage
Here’s how simple it is to generate and use ULIDs with ByteAether.Ulid:
using System;
class Program
{
static void Main()
{
// Create a new ULID
var ulid = Ulid.New();
// Convert to string and back
string ulidString = ulid.ToString();
var ulidFromString = Ulid.Parse(ulidString);
Console.WriteLine($"Generated ULID: {ulid}");
}
}
For detailed usage instructions and a complete API reference, check out the GitHub repository.
Performance Highlights
ByteAether.Ulid outpaces competitors while maintaining strict adherence to the ULID specification.
Library | Generation Speed | Compliance | Error Handling |
---|---|---|---|
ByteAether.Ulid | ✅ Fastest | ✅ Full | ✅ Graceful |
NetUlid | ⚠️ Slower | ✅ Full | ⚠️ Limited |
NUlid | ⚠️ Slower | ✅ Full | ⚠️ Limited |
Cysharp.Ulid | ⚠️ Fast, Non-secure, Non-monotonic | ❌ Incomplete | ⚠️ Limited |
For a full breakdown of benchmarks and testing methodology, visit our GitHub repository.
Open Source and Contributions
ByteAether.Ulid is fully open-source and licensed under the MIT License. Contributions are welcome! Whether you want to report bugs, suggest features, or submit a pull request, we’d love your input.
👉 Submit an Issue
👉 Contribute Code
What’s Next?
Stay tuned for deep dives into:
- Why ULIDs are a game-changer for modern databases.
- Optimizing performance in distributed systems with ULIDs.
- Real-world use cases for ULIDs in .NET applications.
Start simplifying your .NET projects today. Install ByteAether.Ulid and experience the difference!